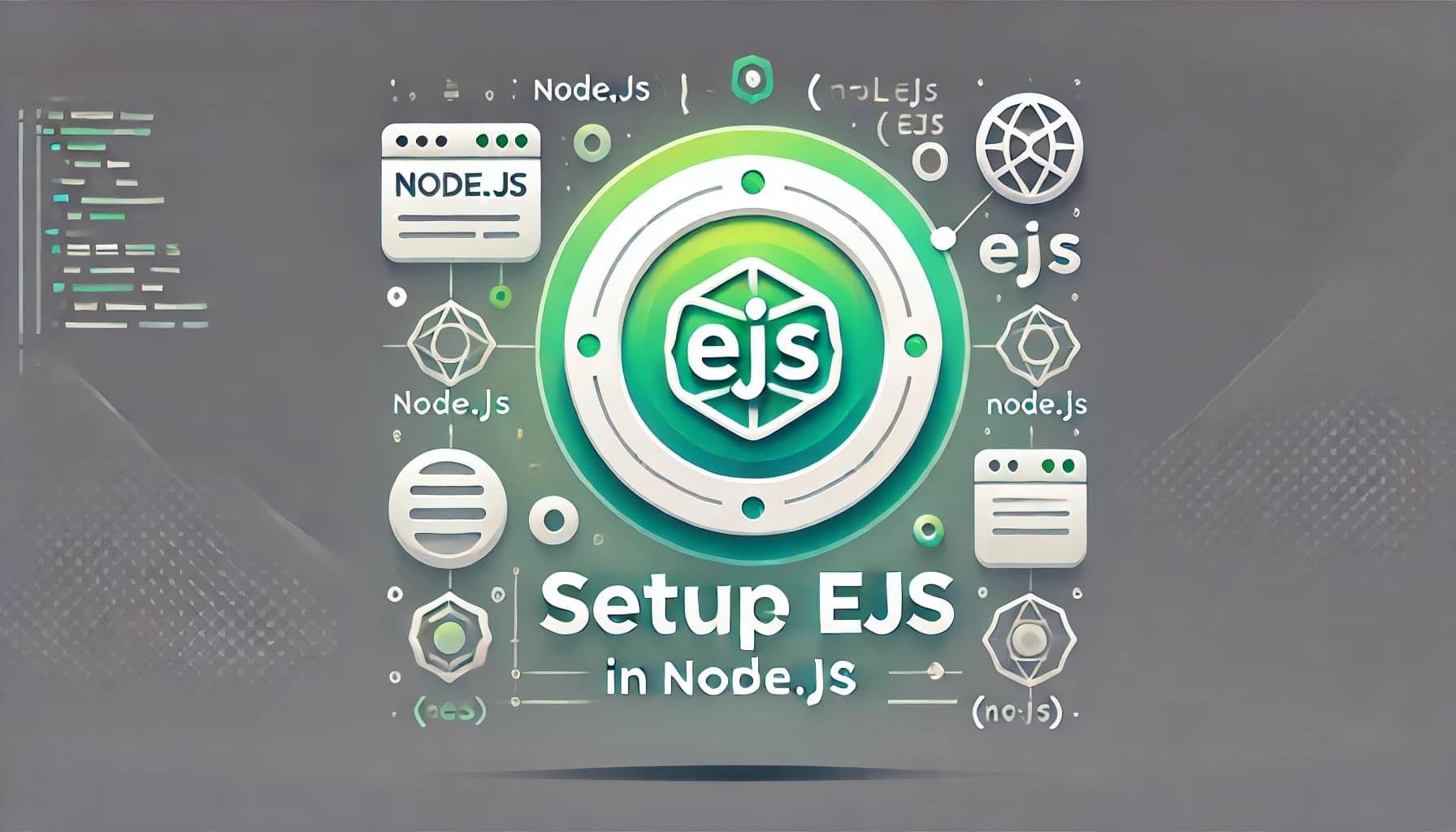
How to Set Up and Use EJS Template Engine in Node.js
EJS (Embedded JavaScript) is a versatile and beginner-friendly templating engine for Node.js, allowing developers to seamlessly embed JavaScript into HTML. In this blog by DeathCode, explore what EJS is, why it’s a go-to choice for dynamic web applications, its alternatives, and a comprehensive step-by-step guide to setting up and using EJS with practical examples, including loops and conditions.
Death Code - 1/20/2025, 6:08:40 AM
Complete Guide to EJS Template Engine
By DeathCode
What is EJS?
EJS (Embedded JavaScript) is a simple templating language that lets you generate HTML markup with plain JavaScript. It is designed to work seamlessly with Node.js, making it a popular choice for building dynamic web applications. With EJS, you can embed JavaScript code directly within your HTML templates, enabling you to create reusable and dynamic content effortlessly.
Why Use EJS?
- Easy to learn and use for developers familiar with JavaScript.
- Supports embedding JavaScript code directly into HTML.
- Allows partials and reusable components for efficient development.
- Integrates seamlessly with Node.js applications.
- Offers flexibility to create dynamic and server-rendered pages.
Alternatives to EJS
While EJS is a great templating engine, there are other alternatives you might consider:
- Pug: Known for its clean syntax and indentation-based structure.
- Handlebars: Focuses on simplicity and logic-less templates.
- Mustache: A minimalist templating language with no logic.
- React/JSX: A component-based approach for building user interfaces.
Step-by-Step Setup of EJS in Node.js
1. Initialize Your Project
mkdir ejs-example
cd ejs-example
npm init -y
2. Install Required Packages
Install Express and EJS:
npm install express ejs
3. Create the Directory Structure
Organize your project as follows:
ejs-example/
├── views/
│ ├── index.ejs
│ └── about.ejs
├── public/
│ └── style.css
├── app.js
├── package.json
4. Set Up the Server
Create app.js
and configure Express to use EJS:
const express = require('express');
const path = require('path');
const app = express();
// Set EJS as the template engine
app.set('view engine', 'ejs');
// Set the views directory
app.set('views', path.resolve('./views'));
// Serve static files
app.use(express.static('public'));
// Routes
app.get('/', (req, res) => {
res.render('index', { title: 'Home', message: 'Welcome to DeathCode EJS Tutorial!' });
});
app.get('/about', (req, res) => {
res.render('about', { title: 'About', description: 'This is the about page created by DeathCode.' });
});
// Start the server
const PORT = 3000;
app.listen(PORT, () => {
console.log(`Server is running on http://localhost:${PORT}`);
});
5. Create EJS Templates
Create index.ejs
:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title><%= title %></title>
</head>
<body>
<h1><%= message %></h1>
<a href="/about">Go to About</a>
</body>
</html>
Create about.ejs
:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title><%= title %></title>
</head>
<body>
<h1>About Page</h1>
<p><%= description %></p>
<a href="/">Go to Home</a>
</body>
</html>
6. Using Loops and Conditions in EJS
You can use JavaScript loops and conditions directly in EJS templates. For example:
<ul>
<% const fruits = ['Apple', 'Banana', 'Cherry']; %>
<% fruits.forEach(fruit => { %>
<li><%= fruit %></li>
<% }); %>
</ul>
Using a condition:
<% if (message) { %>
<p><%= message %></p>
<% } else { %>
<p>No message available.</p>
<% } %>
Conclusion
EJS is a powerful and easy-to-use templating engine that simplifies the process of creating dynamic web pages in Node.js. Whether you are a beginner or an experienced developer, EJS can help you build efficient and maintainable web applications. For more tutorials and tips, stay tuned to DeathCode!