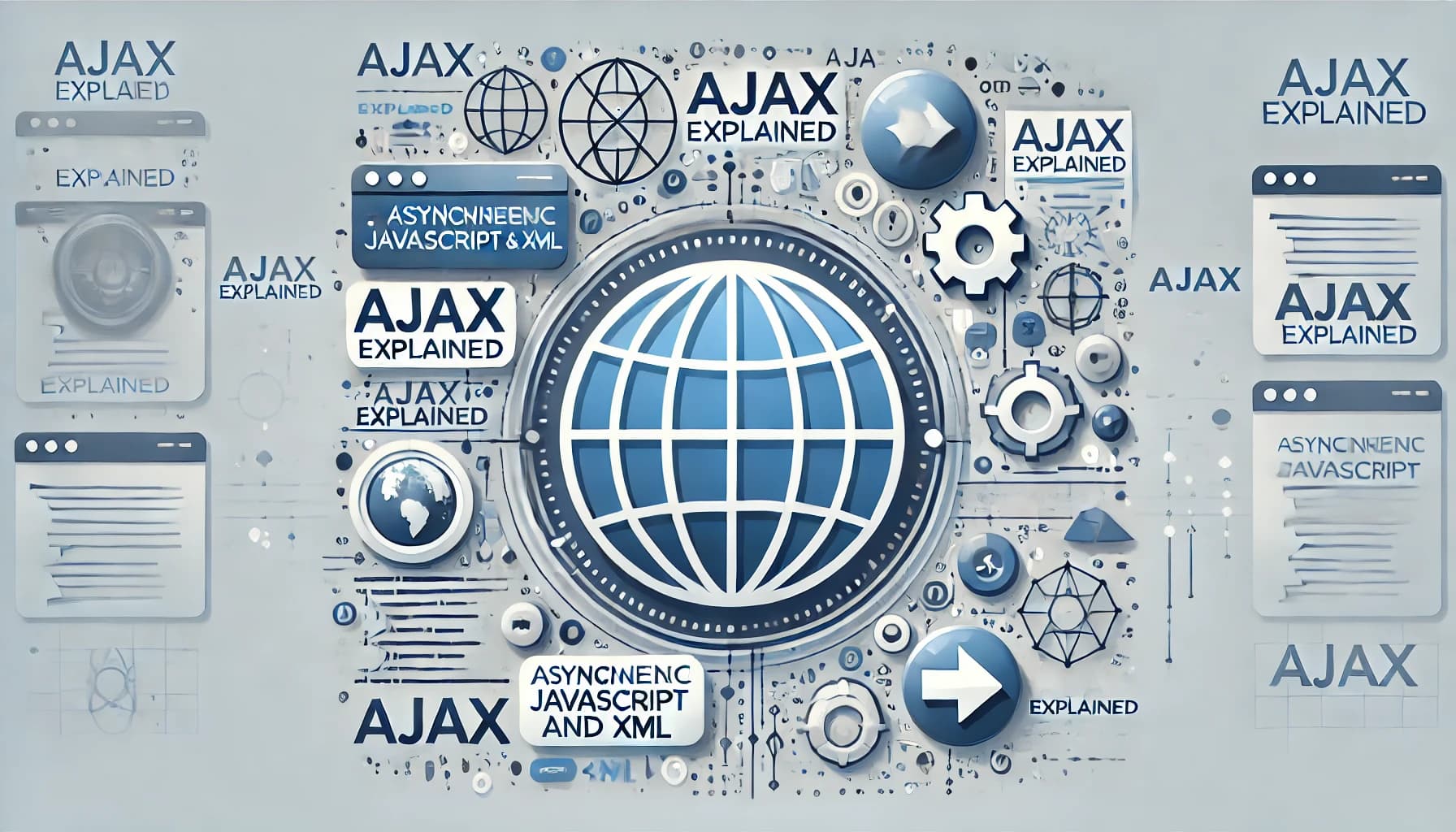
Understanding AJAX: A Comprehensive Guide
AJAX (Asynchronous JavaScript and XML) is a powerful web development technique that enables seamless communication between the client and server without requiring a full page reload. By using AJAX, developers can create dynamic, interactive web applications that update content in real-time, improving user experience and performance. This approach works by sending asynchronous requests to the server, fetching data, and updating specific parts of the webpage, all behind the scenes. Modern implementations often use JSON instead of XML, making AJAX an essential tool for building fast and responsive web applications.
Death Code - 1/19/2025, 12:27:41 PM
Understanding AJAX: A Comprehensive Guide
By Death Code
What is AJAX?
AJAX stands for Asynchronous JavaScript and XML. It is a set of web development techniques that allows a web page to communicate with a server asynchronously, meaning that it can send and receive data without having to reload the entire page. This leads to a more dynamic and responsive user experience.
Why Use AJAX?
AJAX is used to enhance the user experience on web applications. Here are some reasons why AJAX is beneficial:
- Improved Performance: By only updating parts of a web page, AJAX reduces the amount of data transferred between the client and server, leading to faster load times.
- Enhanced User Experience: Users can interact with the application without interruptions, as AJAX allows for seamless updates to the content.
- Reduced Server Load: Since only necessary data is sent and received, AJAX can help reduce the load on the server.
- Dynamic Content: AJAX enables the loading of new content without refreshing the page, allowing for a more interactive experience.
History of AJAX
AJAX was coined in 2005 by Jesse James Garrett in his article titled "Ajax: A New Approach to Web Applications." However, the techniques that make up AJAX have been around for much longer. The XMLHttpRequest object, which is central to AJAX, was introduced by Microsoft in Internet Explorer 5 in 1999. Over the years, AJAX has evolved and become a standard part of web development.
Who Created AJAX?
While Jesse James Garrett popularized the term "AJAX," the underlying technologies were developed by various individuals and organizations. The XMLHttpRequest object was created by Microsoft, and the use of JavaScript for asynchronous communication was pioneered by several developers in the early 2000s.
What Problems Does AJAX Solve?
AJAX addresses several issues in traditional web applications:
- Page Reloads: Traditional web applications require full page reloads to update content, which can be slow and disrupt the user experience. AJAX allows for partial updates, making applications feel faster and more responsive.
- Data Transfer: AJAX minimizes the amount of data transferred between the client and server, which is especially important for mobile users with limited bandwidth.
- Interactivity: AJAX enables developers to create more interactive applications, such as real-time chat applications, live search suggestions, and dynamic forms.
How to Start Using AJAX
To start using AJAX, you need to understand the basic components involved:
- XMLHttpRequest: This is the core object used to send and receive data asynchronously.
- JavaScript: You will use JavaScript to create and manage the XMLHttpRequest object.
- Server-Side Language: You will need a server-side language (like PHP, Node.js, Python, etc.) to handle the requests and send back data.
Basic Example of AJAX
Here’s a simple example of how to use AJAX to fetch data from a server:
// Create a new XMLHttpRequest object
var xhr = new XMLHttpRequest();
// Configure it: GET-request for the URL /data
xhr.open('GET', '/data', true);
// Set up a function to handle the response
xhr.onload = function() {
if ( xhr.status >= 200 && xhr.status < 300) {
// Success! Parse the JSON response
var response = JSON.parse(xhr.responseText);
console.log(response); // Output the response to the console
} else {
console.error('Request failed with status: ' + xhr.status);
}
};
// Send the request
xhr.send();
In this example, we create a new XMLHttpRequest object, configure it to make a GET request to the server at the URL "/data", and define a function to handle the response. When the request is complete, we check the status and log the response to the console if successful.
Real-Life Use Cases of AJAX
AJAX is widely used in modern web applications. Here are some real-life examples:
- Google Maps: When you pan or zoom in Google Maps, AJAX is used to load new map tiles without refreshing the entire page.
- Facebook: Facebook uses AJAX for features like real-time notifications and chat, allowing users to interact without page reloads.
- Online Forms: Many online forms use AJAX to validate user input in real-time, providing instant feedback without submitting the form.
Non-Real-Life Use Cases of AJAX
AJAX can also be used in scenarios that are not directly tied to user interaction:
- Data Fetching: Applications can use AJAX to periodically fetch data from the server, such as stock prices or weather updates, without user intervention.
- Background Sync: AJAX can be used to synchronize data in the background, ensuring that the application has the latest information without disrupting the user experience.
Advantages of Using AJAX
AJAX offers several advantages for web development:
- Faster Load Times: By only loading necessary data, AJAX can significantly reduce load times and improve performance.
- Better User Experience: Users can interact with the application without interruptions, leading to a smoother experience.
- Reduced Server Load: AJAX can help minimize server load by only sending and receiving essential data.
- Increased Interactivity: AJAX allows developers to create more dynamic and interactive applications, enhancing user engagement.
Challenges of Using AJAX
While AJAX has many benefits, it also comes with challenges:
- Browser Compatibility: Different browsers may handle AJAX requests differently, requiring developers to test across multiple platforms.
- SEO Considerations: AJAX content may not be indexed by search engines, which can impact SEO if not handled properly.
- Complexity: Implementing AJAX can add complexity to the codebase, especially when managing multiple asynchronous requests.
Conclusion
AJAX is a powerful tool for modern web development, enabling developers to create dynamic, responsive applications that enhance user experience. By understanding how AJAX works and its various use cases, developers can leverage its capabilities to build efficient web applications. Whether you're creating a simple form or a complex web application, AJAX can help you deliver a seamless experience to your users.
Thank you for reading this comprehensive guide on AJAX! Stay tuned for more tutorials and insights on web development from Death Code.